# Understanding Laravel Queues: A Deep Dive into Background Processing
Written on
Introduction to Laravel Queues
When utilizing a web framework like Laravel, which stands out as one of the most popular PHP frameworks available, developers often encounter lengthy tasks such as data parsing, storage, and uploads during typical web requests. Fortunately, Laravel offers a solution through the creation of queue jobs, enabling these intensive processes to occur in the background. This effectively allows the application to respond more swiftly, as it does not need to complete these tasks immediately.
Laravel supports a standardized API that works with various queue backends, including Amazon SQS, Redis, Beanstalk, synchronous operations, and relational databases.
Setup and Prerequisites
Before diving into queue implementation, it’s essential to familiarize ourselves with the configurations and their purposes.
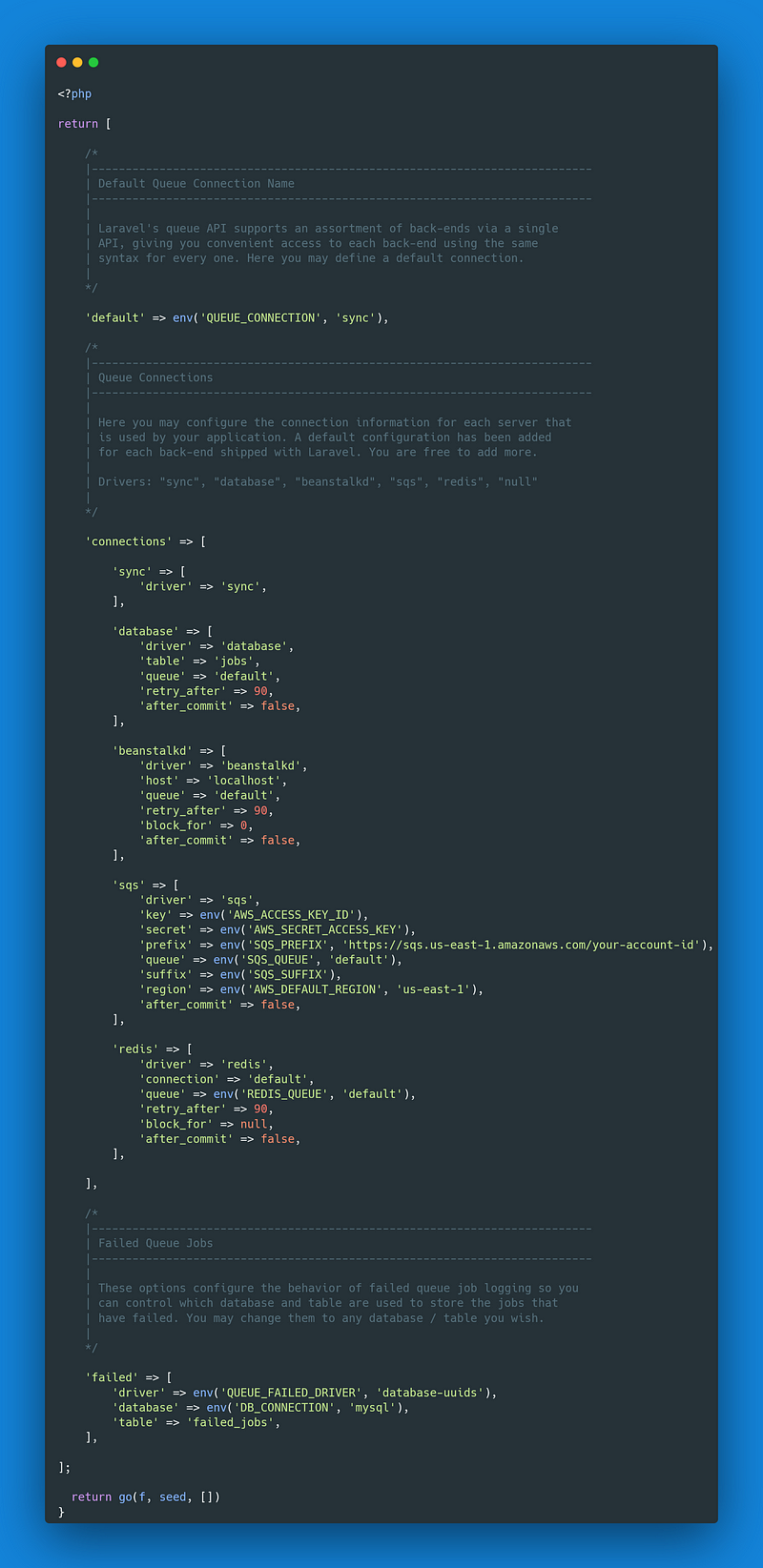
Understanding config/queue.php
The configuration file, config/queue.php, may appear extensive, but we can simplify it into manageable components. The main function of this configuration is to return a key-value array of settings. The default property attempts to retrieve the QUEUE_CONNECTION variable from the .env file; if it's absent, it defaults to synchronous processing, meaning any dispatched job will be executed immediately.
The connections property outlines an array of potential connections for dispatching jobs, which will be elaborated upon in upcoming articles. Typically, smaller applications utilize the database driver. To implement this, we need to create a migration for the jobs table with the following commands:
php artisan queue:table // This command creates the jobs table
php artisan migrate:fresh // This command migrates all the tables
The failed property designates where unsuccessful jobs will be recorded, requiring a database and table as parameters. The corresponding database table is automatically generated upon executing the migration.
Creating Jobs and Basic Configuration
By default, all queues within your application are stored in the app/Jobs directory, which will be created if it does not already exist when you execute the make:job command:
php artisan make:job ProcessPayment
The newly created app/Jobs/ProcessPayment.php file will be structured as follows:
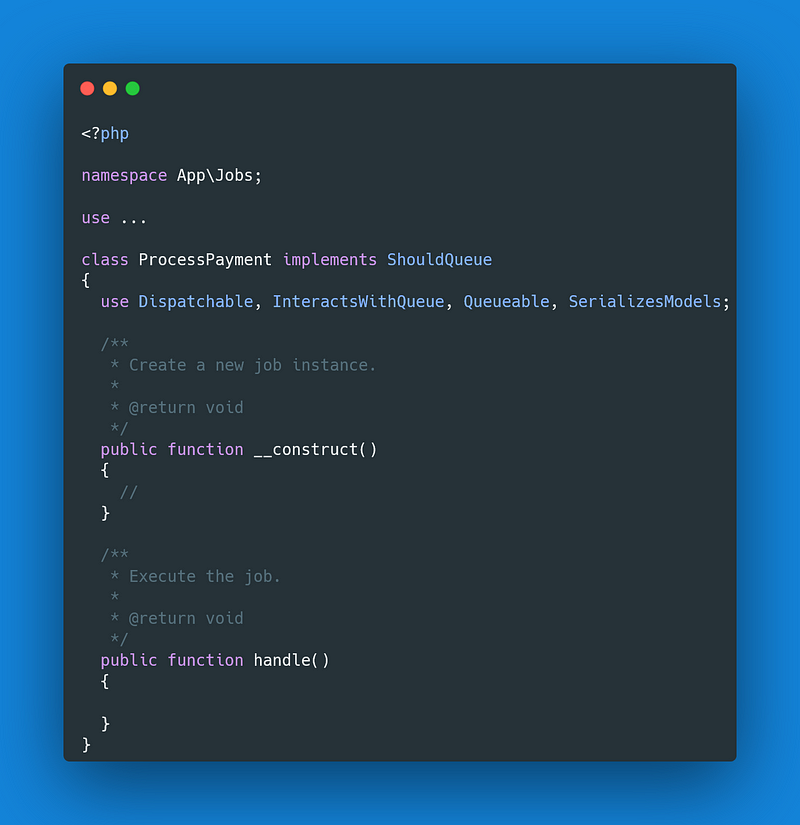
Customizing Job Behavior
You can modify how your job operates by defining various properties. The timeout property accepts an integer that specifies the number of seconds before the worker running the job will terminate it. If the job exceeds this duration, it will fail. This feature is particularly useful to prevent jobs from hanging when dealing with unresponsive third-party applications or libraries.
Additionally, you can set the number of retries; by default, this is zero. By assigning a positive integer value to the $tries property, you can inform the worker how many times to attempt the job before considering it failed. The retryUntil() method further allows you to dictate a time frame during which the job can be retried until it is deemed unsuccessful.
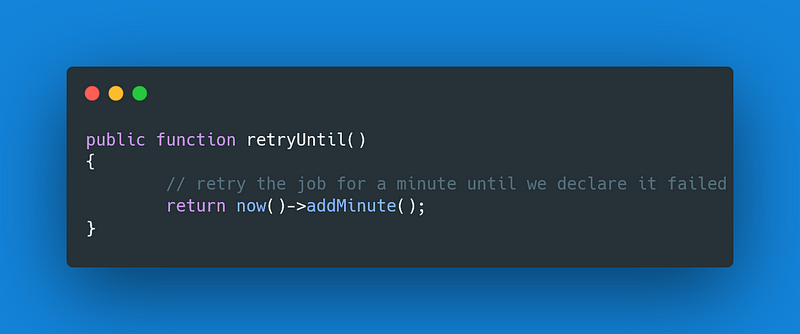
To manage excessive retries within the defined period, you can specify the $backoff property, which takes a positive integer indicating the seconds to wait between each retry.
Upcoming Articles in This Series
Stay tuned for further exploration of Laravel queues with the following articles:
- Laravel Queue — Dispatching Workflows Basics
- Laravel Queue — Dispatching Workflows Advanced