# Simplifying Transfer Learning with PyTorch's New Multi-Weight API
Written on
Chapter 1: Introduction to Fine-Tuning with PyTorch
Fine-tuning deep learning (DL) models has become exceedingly easy thanks to contemporary frameworks like TensorFlow and PyTorch. In just a few minutes, you can adapt a well-established artificial neural network to suit your specific requirements.
However, fine-tuning is merely one aspect of the experiment process. Typically, the workflow follows these steps:
- Select and instantiate the neural network architecture you wish to modify. For instance, if you're focusing on computer vision tasks, you might choose the ResNet architecture.
- Load a predefined set of weights for this architecture. In the context of computer vision, weights trained on the ImageNet dataset are often preferred.
- Create a preprocessing function or a series of operations to format your data appropriately.
- Train the neural network you've set up on your data. You may need to adjust the output layer to fit your needs or freeze certain layers to maintain a portion of your weights. These choices depend on your specific application.
- Assess your trained model using a reserved test dataset. Ensure that the test data is processed in the same manner as the training data; even minor inconsistencies can negatively affect performance and be difficult to diagnose.
- Record the metadata of your experiment, such as the names of the dataset classes, for future applications or sanity checks.
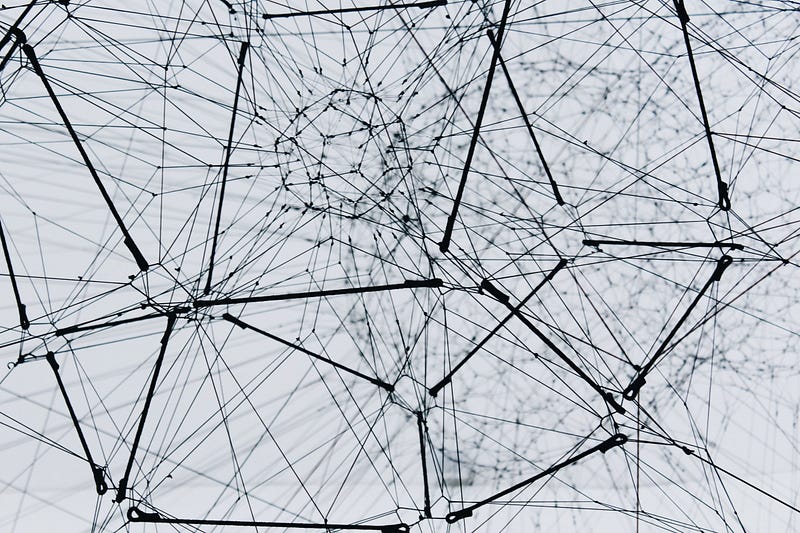
Chapter 2: The Old Methodology
At this point, one might think, "This isn't as straightforward as it seems!" You're correct; the intricacies involved can be daunting if you're handling every detail manually. Let’s explore how the new PyTorch API simplifies this process, saving you both time and effort.
For a deeper understanding, let’s first look at traditional approaches. While we won’t be training a model, we will cover nearly all other aspects:
- Load a pre-trained neural network architecture.
- Preprocess the dataset.
- Utilize the neural network to make predictions on a test set.
- Use your dataset's metadata to generate a human-readable outcome.
The following code snippet encapsulates the necessary steps to meet the above criteria:
# Load ResNet architecture with pre-trained weights
model = resnet50(pretrained=True)
# Define and initialize data transformations
transform = transforms.Compose([
transforms.Resize(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# Process the image and pass it through the network
output = model(processed_image)
In this code, you first load the ResNet architecture with the pretrained flag set to True, instructing PyTorch to use pre-trained weights rather than initializing them randomly. You then specify a series of transformations to preprocess the image before passing it through the model.
Finally, you want to present the results in a way that's easily understandable. Instead of merely indicating that the model predicts class 4, you aim for clarity, such as stating that the model believes the image depicts a dog with 95% confidence. This requires you to load a metadata file that contains the class names to match predictions accurately.
While this script is relatively simple, it has two notable shortcomings:
- A small change in how you process your test dataset could result in hard-to-trace errors. It's vital to ensure that both training and test datasets undergo the same transformations; otherwise, discrepancies can lead to performance issues.
- You must keep a metadata file accessible at all times. Any alterations to this file could yield unexpected results, leading to frustrations.
Chapter 3: Leveraging the New PyTorch API
Now, let’s explore how the new PyTorch API enhances this workflow.
With the newly introduced API, you can address two main challenges: ensuring consistent processing between training and testing subsets, and eliminating the need for a separate metadata file.
Here’s an example illustrating these improvements:
# Simplified script with new PyTorch API
model = resnet50(weights=ResNet50_Weights.ImageNet1K_V2)
Notice how the script is significantly more concise. This reduction in length stems from not needing to define a preprocessing function or manage a separate metadata file.
In this new approach, you create a weights object first, which contains both the transformations applied during training and the dataset's metadata. This integration is a significant advancement!
You also have more flexibility in selecting which weights to preload. For instance, if you want to load a different set of weights that achieved an 80.674% accuracy, you simply modify your code as follows:
model = resnet50(weights=ResNet50_Weights.ImageNet1K_V2)
Or you can opt for the weights that provided the best results on ImageNet with:
model = resnet50(weights=ResNet50_Weights.default)
Conclusion
The process of fine-tuning deep learning models has never been easier, thanks to modern frameworks like TensorFlow and PyTorch. However, it’s essential to avoid common pitfalls, such as ensuring consistent processing for training and test subsets, and the need to manage separate metadata files.
Moreover, consider what happens if you require a different set of weights or want to share weights through a centralized repository. The new multi-weight support API in PyTorch effectively addresses these issues, allowing for smoother experimentation. If you’re interested, you can try it out by installing the nightly version of PyTorch and provide your feedback on this GitHub issue.
About the Author
I'm Dimitris Poulopoulos, a machine learning engineer at Arrikto. I have developed AI and software solutions for prominent clients, including the European Commission, Eurostat, IMF, the European Central Bank, OECD, and IKEA. For more insights into Machine Learning, Deep Learning, Data Science, and DataOps, feel free to connect with me on Medium, LinkedIn, or Twitter @james2pl. Please note that my views are my own and do not reflect those of my employer.