Mastering JavaScript Array Filtering: A Comprehensive Guide
Written on
Chapter 1: Introduction to Array Filtering
In JavaScript, manipulating arrays is a fundamental task, and one of the most effective methods at your disposal is array filtering. Whether you're creating a dynamic web application, processing data, or managing user inputs, mastering the ability to filter arrays can significantly streamline your workflow.
This article delves into the nuances of filtering arrays in JavaScript, highlighting a variety of methods and techniques to help you excel in this critical skill.
Section 1.1: Understanding the filter() Method
The filter() method is a built-in functionality that generates a new array composed of elements that meet a specified condition defined by a provided function. It is an invaluable tool for extracting a subset of elements from an array based on particular criteria.
For example:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers = numbers.filter(function(number) {
return number % 2 === 0;
});
console.log(evenNumbers); // Output: [2, 4, 6, 8, 10]
In this snippet, an array of numbers is created. The filter() method is then employed to produce a new array called evenNumbers, which includes only the even numbers from the original array. The filter() method executes a callback function for each element, including the element in the new array if the callback returns true.
For a more concise syntax, you can utilize arrow functions:
const fruits = ['apple', 'banana', 'orange', 'pear', 'kiwi'];
const longFruits = fruits.filter(fruit => fruit.length > 5);
console.log(longFruits); // Output: ['banana', 'orange']
Here, we filter an array of fruits to form a new array called longFruits, which contains only the fruits with names longer than five characters.
Subsection 1.1.1: Exploring every() and some() Methods
While primarily not used for filtering, the every() and some() methods are also beneficial when working with arrays.
The every() method checks if all elements in an array fulfill a specific condition, whereas the some() method verifies if at least one element meets a specific criterion.
const ages = [18, 22, 30, 15, 27];
const allAdults = ages.every(age => age >= 18);
const hasMinor = ages.some(age => age < 18);
console.log(allAdults); // Output: false
console.log(hasMinor); // Output: true
In this example, the every() method determines whether all ages in the array are 18 or older (allAdults), while the some() method checks if there is at least one age below 18 (hasMinor).
Section 1.2: Advanced Filtering Techniques
Although the filter() method is powerful on its own, combining it with additional techniques, such as object destructuring and the spread operator, can yield even more sophisticated filtering solutions.
const people = [
{ name: 'Alice', age: 25, city: 'New York' },
{ name: 'Bob', age: 30, city: 'Chicago' },
{ name: 'Charlie', age: 35, city: 'New York' },
{ name: 'David', age: 40, city: 'Chicago' }
];
const newYorkers = people.filter(({ city }) => city === 'New York');
const adults = [...people.filter(({ age }) => age >= 18)];
console.log(newYorkers); // Output: [{ name: 'Alice', age: 25, city: 'New York' }, { name: 'Charlie', age: 35, city: 'New York' }]
console.log(adults); // Output: [{ name: 'Alice', age: 25, city: 'New York' }, { name: 'Bob', age: 30, city: 'Chicago' }, { name: 'Charlie', age: 35, city: 'New York' }, { name: 'David', age: 40, city: 'Chicago' }]
In this illustration, we use object destructuring to filter an array of people based on their city and age. The new array, newYorkers, contains only individuals living in New York, while the adults array includes all individuals aged 18 and above. The spread operator is also employed to create a new array from the filtered output.
Chapter 2: Conclusion
In conclusion, filtering arrays in JavaScript is a potent technique that can significantly improve your data manipulation capabilities. Whether dealing with simple arrays of numbers or more complex objects, mastering the filter() method, along with related methods like every() and some(), equips you with the necessary tools to extract relevant information efficiently.
By merging these methods with advanced techniques like object destructuring and the spread operator, you can develop more elegant and powerful solutions. Start experimenting with array filtering in JavaScript, and you'll quickly become proficient at manipulating data.
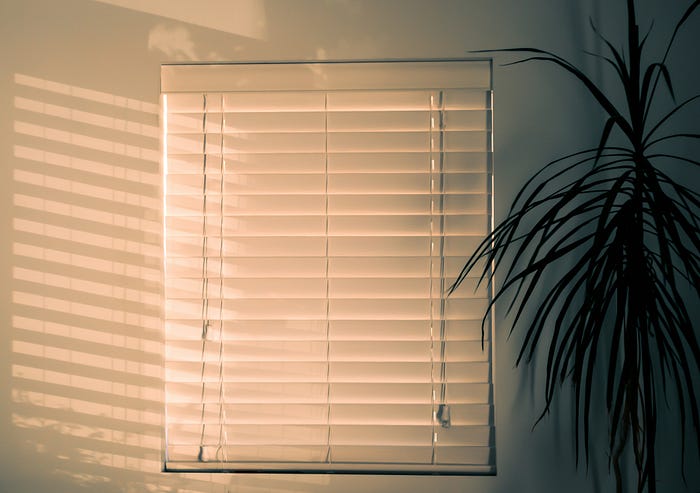
This video titled "JavaScript Array Mastery: Tips, Tricks & Best Practices" provides insights into optimizing your array handling skills in JavaScript.
The video "JavaScript Filter Function" explores the filter method and its practical applications, helping you enhance your array manipulation techniques.