Finding Min and Max Values in Arrays with JavaScript
Written on
Understanding the Problem
In today's coding challenge, we will tackle the task of determining the minimum and maximum values from a series of numbers provided as a space-separated string. The challenge is to return both values in a specific format.
For instance:
- Calling highAndLow("1 2 3 4 5") should yield "5 1".
- For highAndLow("1 2 -3 4 5"), the output will be "5 -3".
- And for highAndLow("1 9 3 4 -5"), the result is "9 -5".
This exercise is an excellent way to practice string manipulation and array handling.
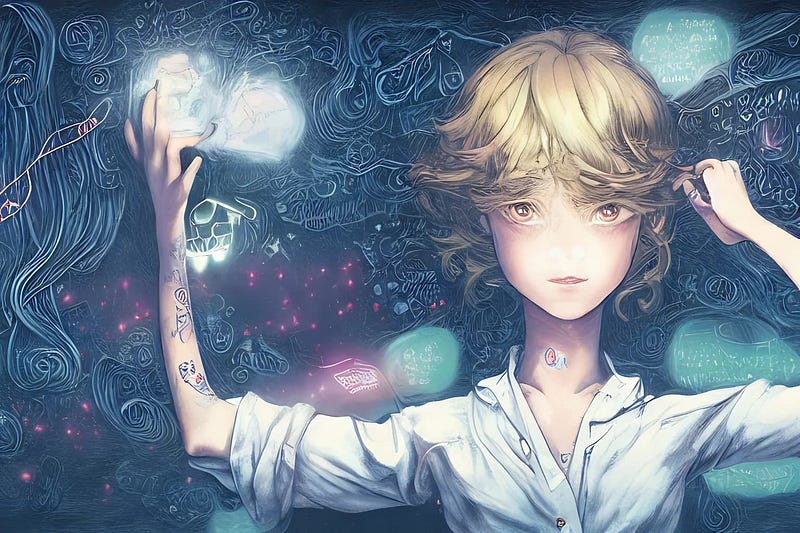
My Approach to the Solution
Here's how I approached the problem using TypeScript:
export class Kata {
static highAndLow(numbers: string): string {
const arr: number[] = numbers.split(" ").map((c) => parseInt(c));
const max: number = Math.max(...arr);
const min: number = Math.min(...arr);
return ${max} ${min};
}
}
In JavaScript, the solution looks like this:
function highAndLow(numbers) {
const arr = numbers.split(" ").map((c) => parseInt(c));
const max = Math.max(...arr);
const min = Math.min(...arr);
return ${max} ${min};
}
I opted to break the problem down into clear steps. Initially, I split the input string into an array of numbers. Next, I utilized the built-in Math.max and Math.min functions to derive the highest and lowest values, respectively. Finally, I formatted and returned the result as a string.
Breaking Down the Steps
To split the input string, I used the String.split() method, which generates an array of strings.
const arrString: string[] = numbers.split(" ");
In JavaScript, this is done similarly:
const arrString = numbers.split(" ");
Next, I converted each string in the array to a number using the Array.map() method:
const arrNumber: number[] = arrString.map((c) => parseInt(c));
In JavaScript:
const arrNumber = arrString.map((c) => +c);
After that, I used Math.max() and Math.min() to find the maximum and minimum values:
const max: number = Math.max(...arr);
const min: number = Math.min(...arr);
And in JavaScript:
const max = Math.max(...arr);
const min = Math.min(...arr);
An Alternative Method
Another way to solve this problem is by sorting the array after converting the string to numbers. You can then return the first and last elements as shown here:
export class Kata {
static highAndLow(numbers: string) {
let arr = numbers
.split(" ")
.map((x) => parseInt(x))
.sort((a, b) => a - b);
return ${arr[arr.length - 1]} ${arr[0]};
}
}
In JavaScript, this would be:
function highAndLow(numbers) {
const arr = numbers
.split(" ")
.map((c) => parseInt(c))
.sort((a, b) => a - b);
return ${arr[arr.length - 1]} ${arr[0]};
}
Conclusion
Thank you for reading! I hope this explanation helps you better understand how to find the minimum and maximum values in an array using JavaScript and TypeScript. Stay tuned for more coding challenges!
This video demonstrates how to find the minimum and maximum values in an array using JavaScript.
A beginner's guide to finding min and max numbers in an array without using built-in methods.