Demystifying Python: Understanding 10 Complex Concepts
Written on
Chapter 1: Introduction to Complex Python Concepts
Are you prepared to explore the complexities of Python programming? Whether you're an experienced programmer or a beginner, this detailed guide simplifies the ten most challenging Python concepts. You'll find step-by-step explanations and code snippets designed to enhance your understanding. Let’s get started!
Section 1.1: Generators
Generators provide a unique method for creating iterators in Python, enabling the generation of values on-the-fly without storing the entire sequence in memory. To define a generator, use the yield statement within a function. Below is a simple example:
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
for value in gen:
print(value)
Section 1.2: Decorators
Decorators serve to alter the functionality of a function or method. You can create your own decorator by using the @decorator_name syntax. Here’s a basic illustration:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
Subsection 1.2.1: Essential Python Concepts
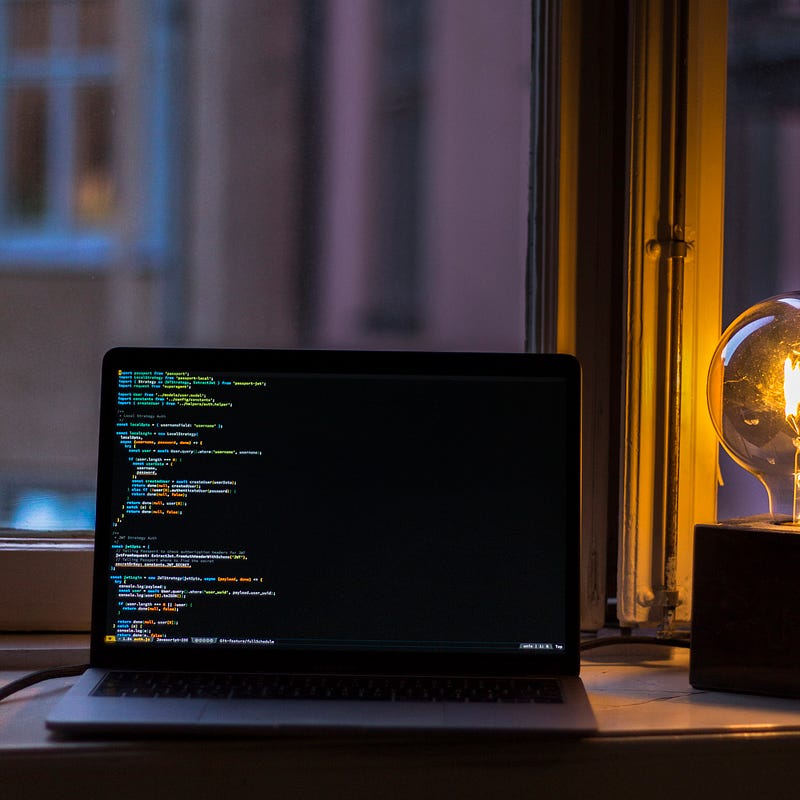
Section 1.3: Context Managers
Context managers are crucial for resource management, implemented using the __enter__ and __exit__ methods. They are particularly effective for managing files and databases. Here’s a file handling example:
with open('example.txt', 'r') as file:
data = file.read()
# Process data as needed
Section 1.4: List Comprehensions
List comprehensions offer a succinct way to construct lists. For instance, here's how to create a list of squares:
squares = [x**2 for x in range(10)]
Chapter 2: Advanced Python Features
This video titled "Python 101: Learn the 5 Must-Know Concepts" dives into essential concepts for Python beginners, providing valuable insights and practical examples.
Section 2.1: Lambda Functions
Lambda functions are compact, unnamed functions defined using the lambda keyword, ideal for short-lived operations. Here’s a straightforward example:
double = lambda x: x * 2
print(double(5))
Section 2.2: Recursion
Recursion occurs when a function calls itself to resolve a problem. Below is a classic example that calculates the factorial of a number:
def factorial(n):
if n == 1:
return 1else:
return n * factorial(n-1)
Section 2.3: Regular Expressions
Regular expressions are powerful for identifying patterns in text. For instance, to check if an email address is valid, you can use the following code:
import re
pattern = r'^[w.-]+@[w.-]+$'
email = '[email protected]'
if re.match(pattern, email):
print("Valid email")
else:
print("Invalid email")
Section 2.4: Concurrency with Threading
Threading allows multiple threads to run concurrently, improving efficiency. Here’s a simple demonstration:
import threading
def print_numbers():
for i in range(1, 6):
print(f"Number {i}")
def print_letters():
for letter in 'abcde':
print(f"Letter {letter}")
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
thread1.start()
thread2.start()
Section 2.5: Object-Oriented Programming (OOP)
OOP is a core principle in Python programming. Below is a basic example of defining a class:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} says Woof!")
my_dog = Dog("Buddy", "Golden Retriever")
my_dog.bark()
Section 2.6: Virtual Environments
Virtual environments are essential for managing project dependencies. Here’s how to create and activate one:
python -m venv myenv
source myenv/bin/activate # On Windows, use myenvScriptsactivate
Wrapping Up
Congratulations! You have explored these intricate Python concepts, and now you have a better grasp of them. Remember, the path to mastery lies in continuous practice and exploration.
We’d love to hear your thoughts on this post! Was it insightful? Did it provide useful programming tips? Feel free to share your feedback!
? FREE E-BOOK ? — Discover our complimentary e-book, available here.
? BREAK INTO TECH + GET HIRED — Interested in entering the tech field? Check out our guide.
If you enjoyed this article and want more, follow us! ? Thank you for being part of our community! Before you leave:
Be sure to clap and follow the writer! ? You can find even more content at PlainEnglish.io ? Sign up for our free weekly newsletter. ?? Follow us on Twitter(X), LinkedIn, YouTube, and Discord.
The second video titled "PLEASE Learn These 10 Advanced Python Features" offers a deeper dive into advanced features that every Python developer should know, enhancing your programming toolkit.